This article is prepared by our Salesforce Developer Sergey Tit.
Securing authentication is crucial as passwords and PINs are easily compromised, leading to an increasing need for safer and more convenient methods. Biometric authentication offers a solution that enhances both security and user experience. To address this need, Salesforce introduced the Lightning Web Component Biometrics Service API, which allows developers to easily add biometric authentication to their applications. This post highlights the features of this API and how it can be used within the Salesforce platform.
Biometric authentication uses unique traits like fingerprints, facial features, iris patterns, or voice to confirm a user's identity. Unlike passwords or tokens, which can be lost or stolen, biometric data is tied to an individual and hard to replicate. This makes it a strong option for securing applications in areas like mobile banking and healthcare.
The BiometricsService API in the Lightning web component allows users to authenticate their identity using their device's biometric features. The results of these actions are sent back to the Lightning web component. Biometric checks are handled locally on the device and don’t need an internet connection. However, the service requires access to platform-specific APIs, which are only available in compatible Salesforce mobile apps.
Biometric authentication uses unique traits like fingerprints, facial features, iris patterns, or voice to confirm a user's identity. Unlike passwords or tokens, which can be lost or stolen, biometric data is tied to an individual and hard to replicate. This makes it a strong option for securing applications in areas like mobile banking and healthcare.
The BiometricsService API in the Lightning web component allows users to authenticate their identity using their device's biometric features. The results of these actions are sent back to the Lightning web component. Biometric checks are handled locally on the device and don’t need an internet connection. However, the service requires access to platform-specific APIs, which are only available in compatible Salesforce mobile apps.
Architecture and Design
- Biometric Module: BiometricsService is a JavaScript module designed to interact with native device biometric hardware, such as fingerprint sensors, facial recognition systems, or iris scanners.
- API Integration: The service integrates with Salesforce Lightning Web Components (LWC) and provides a set of methods to facilitate biometric authentication processes.
- Local Handling: The actual biometric authentication is handled locally on the device, ensuring that sensitive biometric data does not leave the device and is not exposed to external servers.
The benefits of using Salesforce’s Biometric Authentication
- Enhanced Security: Biometric authentication provides an additional layer of protection, making it more difficult for unauthorized users to access the application.
- Improved User Experience: It simplifies the login process, eliminating the need for complex passwords, and offers a more convenient and satisfying experience for users.
Integration steps
Ready to unlock the power of BiometricsService API? Here’s how:
1) Setup and Configuration:
a. Salesforce Mobile App: Ensure that the Salesforce mobile app is correctly set up and supports the latest version of BiometricsService.
b. Mobile Publisher: For custom Experience Cloud sites, ensure that the Mobile Publisher app is configured to support biometric authentication.
2) Import the API: Add import { getBiometricsService } from 'lightning/mobileCapabilities'; to your component's JavaScript file.
1) Setup and Configuration:
a. Salesforce Mobile App: Ensure that the Salesforce mobile app is correctly set up and supports the latest version of BiometricsService.
b. Mobile Publisher: For custom Experience Cloud sites, ensure that the Mobile Publisher app is configured to support biometric authentication.
2) Import the API: Add import { getBiometricsService } from 'lightning/mobileCapabilities'; to your component's JavaScript file.

3) Check Availability: Verify the API is accessible with
getBiometricsService().isAvailable()
const biometricsService = getBiometricsService();
biometricsService.isAvailable().then(isAvailable => {
if (isAvailable) {
// Proceed with biometric operations
}
});
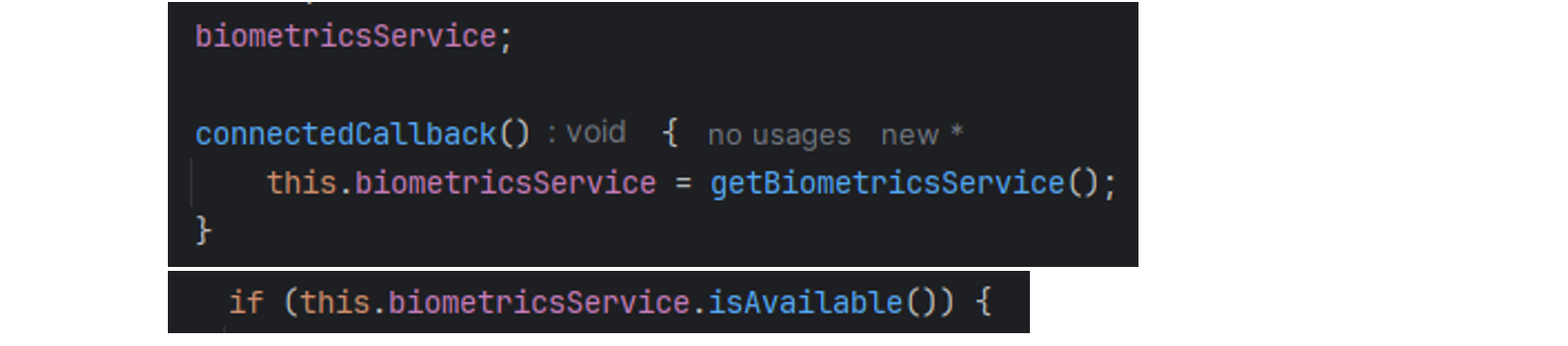
4) Check Biometrics Readiness: Ensure the device has biometric capabilities and is set up with
getBiometricsService().isBiometricsReady(options)
biometricsService.isBiometricsReady(options).then(isReady => {
if (isReady) {
// Device is ready for biometric authentication
}
});

5) Prompt a Biometric Check: Initiate a biometric check with
getBiometricsService().checkUserIsDeviceOwner(options)
biometricsService.checkUserIsDeviceOwner(options).then(isOwner => {
if (isOwner) {
// User authenticated successfully
}
});

6) Handling Errors: Implement error handling for scenarios where biometric authentication might fail due to unsupported devices or incorrect configurations.
Best Practices
- Mobile-Only Features: Ensure that biometric functionalities are only utilized in mobile environments to avoid compatibility issues.
- Fallback Mechanisms: Implement alternative authentication methods for cases where biometric checks are not feasible, such as on desktops or unsupported devices.
- Clear Instructions: Offer clear instructions and feedback to users when biometric authentication is required or fails.
- Cross-Device Testing: Test biometric features across different devices and operating systems to ensure compatibility and performance.
Advanced Use Cases
1) Enhanced Security for Transactions:
a. Financial Apps: Use biometric authentication to authorize financial transactions or access sensitive account information.
b. Healthcare Apps: Secure patient data and medical records with biometric authentication for authorized personnel.
2) Conditional Access:
a. Secured Records: Display sensitive records or features only after successful biometric authentication, ensuring that unauthorized users cannot access this data.
b. Role-Based Access Control: Implement biometric authentication to grant access to different levels of functionality based on user roles.
3) Custom Workflows:
a. Automated Processes: Trigger biometric authentication as part of automated workflows, such as onboarding new users or validating high-stakes actions within the app.
4) Integration with Other Services:
a. Third-Party Apps: Integrate BiometricsService with other third-party authentication services to provide a seamless user experience across platforms.
b. Single Sign-On (SSO): Combine biometric authentication with SSO solutions to streamline access across multiple applications.
a. Financial Apps: Use biometric authentication to authorize financial transactions or access sensitive account information.
b. Healthcare Apps: Secure patient data and medical records with biometric authentication for authorized personnel.
2) Conditional Access:
a. Secured Records: Display sensitive records or features only after successful biometric authentication, ensuring that unauthorized users cannot access this data.
b. Role-Based Access Control: Implement biometric authentication to grant access to different levels of functionality based on user roles.
3) Custom Workflows:
a. Automated Processes: Trigger biometric authentication as part of automated workflows, such as onboarding new users or validating high-stakes actions within the app.
4) Integration with Other Services:
a. Third-Party Apps: Integrate BiometricsService with other third-party authentication services to provide a seamless user experience across platforms.
b. Single Sign-On (SSO): Combine biometric authentication with SSO solutions to streamline access across multiple applications.
BiometricsService API base example
.html
<template>
<lightning-card title="Biometrics Service Demo" icon-name="custom:privately_shared">
<div class="slds-var-m-around_medium">
Use device biometrics capabilities to verify current user is indeed device owner:
<lightning-button
variant="brand"
label="Verify"
title="Verify device ownership using biometrics"
onclick={handleVerifyClick}
class="slds-var-m-left_x-small">
</lightning-button>
</div>
<div class="slds-var-m-around_medium">
<lightning-formatted-text value={status}></lightning-formatted-text>
</div>
</lightning-card>
</template>
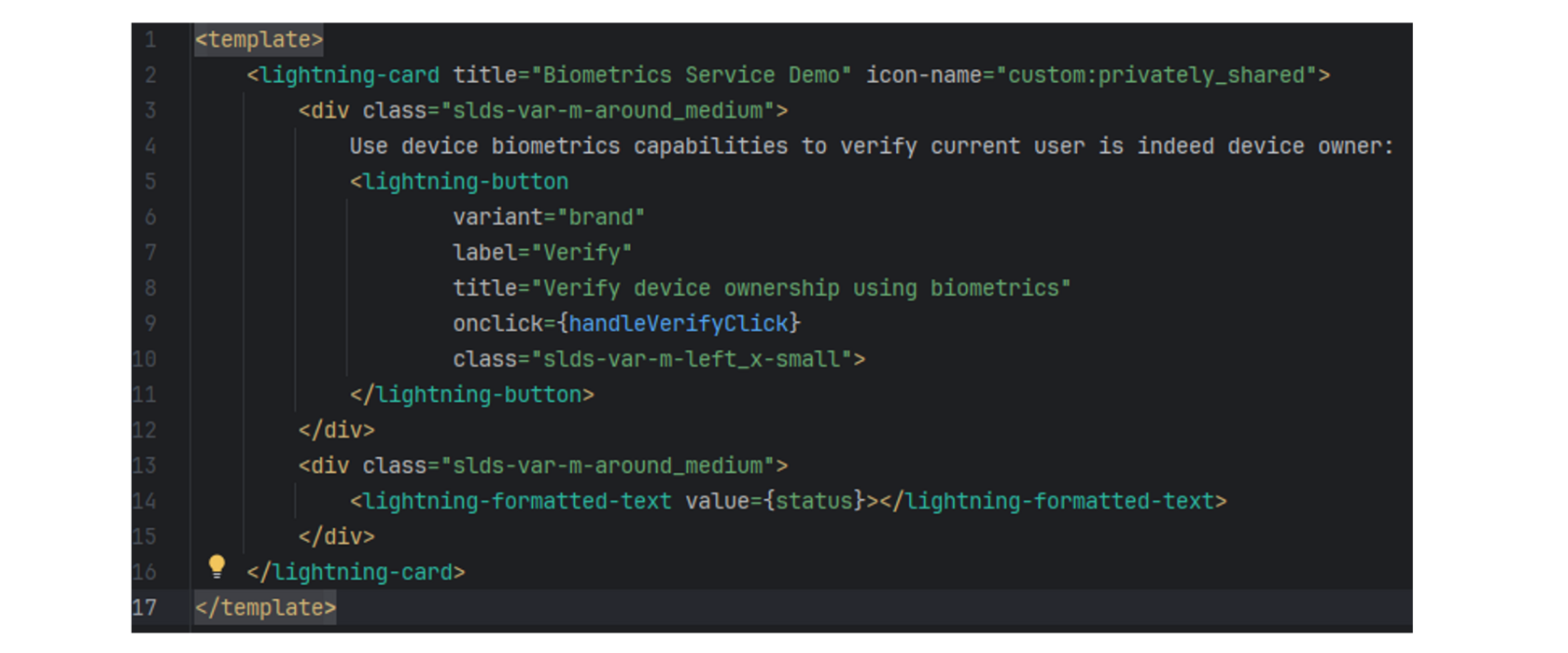
.js
import {LightningElement} from 'lwc';
import {getBiometricsService} from 'lightning/mobileCapabilities';
export default class BioService extends LightningElement {
status;
biometricsService;
connectedCallback() {
this.biometricsService = getBiometricsService();
}
handleVerifyClick() {
if (this.biometricsService.isAvailable()) {
const options = {
permissionRequestBody: "Required to confirm device ownership.",
additionalSupportedPolicies: ['PIN_CODE']
};
this.biometricsService.checkUserIsDeviceOwner(options)
.then((result) => {
// Do something with the result
if (result === true) {
this.status = "✔ Current user is device owner."
} else {
this.status = "𐄂 Current user is NOT device owner."
}
})
.catch((error) => {
// Handle errors
this.status = 'Error code: ' + error.code + '\nError message: ' + error.message;
});
} else {
// service not available
this.status = 'Problem initiating Biometrics service. Are you using a mobile device?';
}
}
}
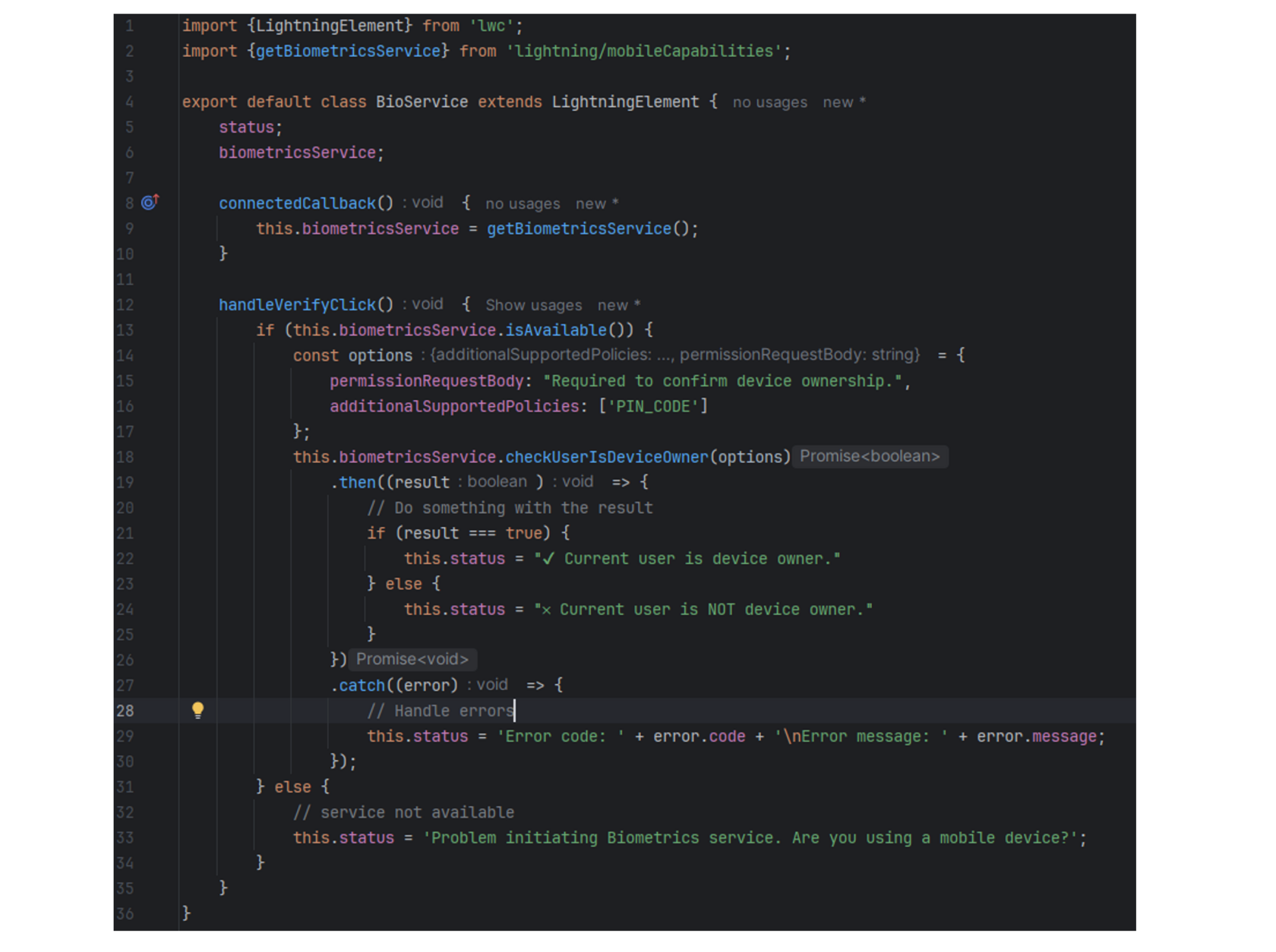
Showroom
Here’s how it works in the Android Salesforce Mobile app: It’s a straightforward Lightning Component with tabs.
Watch the demo:
video_sf_mobile_app_tab.mp4
In the Android Publisher Playground app, it works as a simple Experience page using the same LWC components.
Watch the demo:
video_sd_publisher_playground_app_site.mp4
The BiometricsService is compatible with the Salesforce Mobile App Plus and the Mobile Publisher app, but it does not work with Field Service Mobile.
Watch the demo:
video_sf_mobile_app_tab.mp4
In the Android Publisher Playground app, it works as a simple Experience page using the same LWC components.
Watch the demo:
video_sd_publisher_playground_app_site.mp4
The BiometricsService is compatible with the Salesforce Mobile App Plus and the Mobile Publisher app, but it does not work with Field Service Mobile.
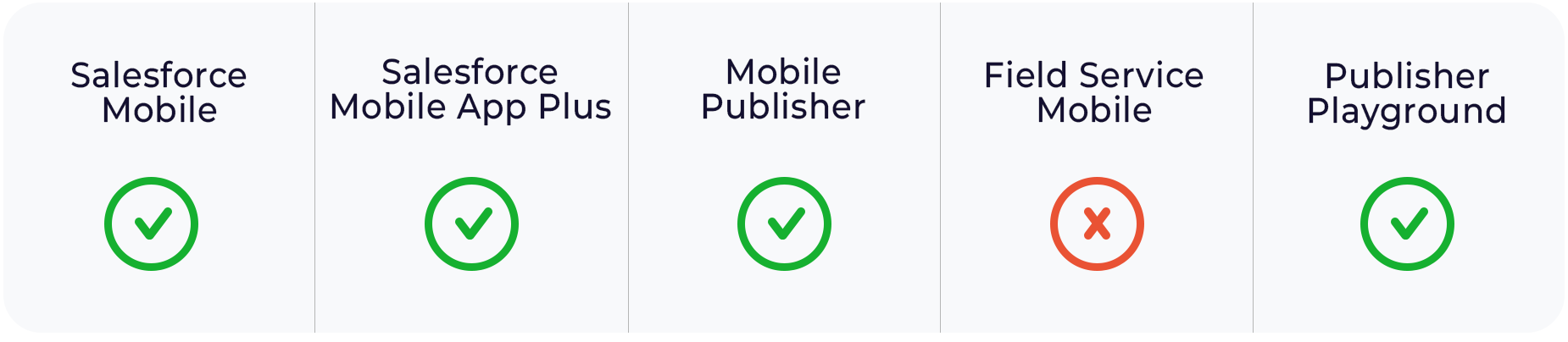
The service is available on iOS and Android apps but is not supported on desktop.
Attempting to use the BiometricsService in the desktop version results in an error.
Watch the demo:
video_sf_desktop_error.mp4
Watch the demo:
video_sf_desktop_error.mp4
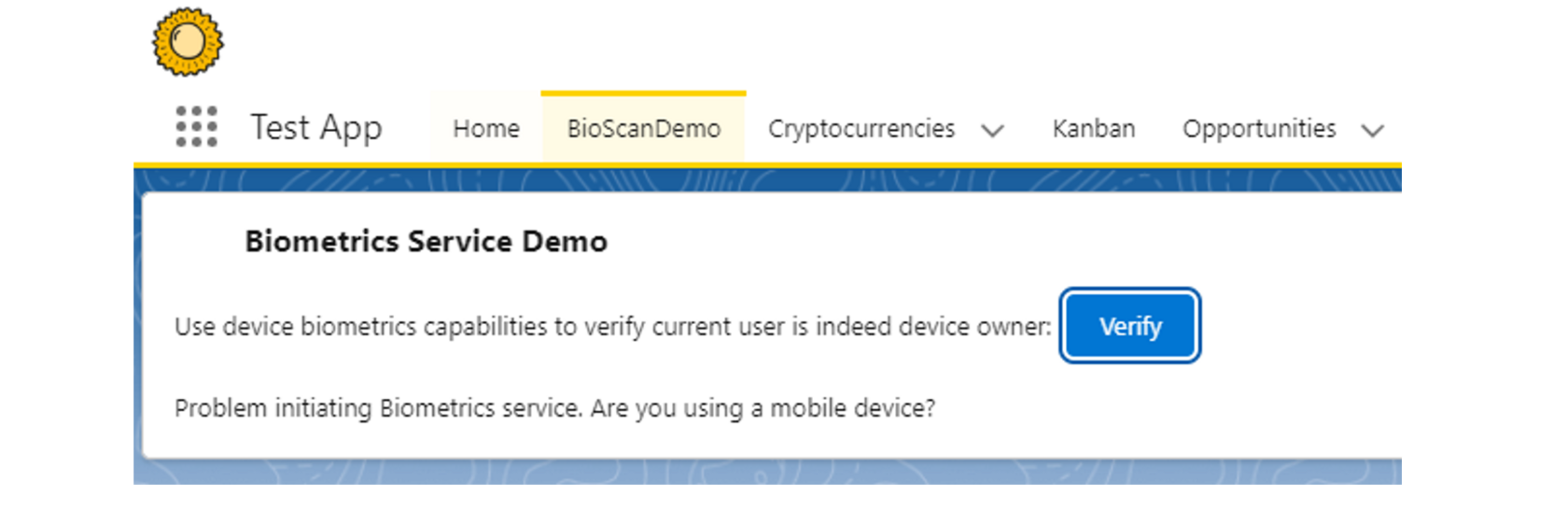
Use case examples
There are many ways to use the Salesforce BiometricsService.
Example 1:
You can use it with a Submit button to update the Status field of an Order record. When a user clicks the Submit button, the BiometricsService runs, and if successful, it updates the Order status. Here's the LWC component and Action button for this setup.
Use this URL for the Submit button:
/lightning/action/quick/Order.Submit_new?context=RECORD_DETAIL&recordId={!CASESAFEID(Order.Id)}.
It retrieves the record ID, opens the 'Submit_new' action, and passes the record ID to it.
Example 1:
You can use it with a Submit button to update the Status field of an Order record. When a user clicks the Submit button, the BiometricsService runs, and if successful, it updates the Order status. Here's the LWC component and Action button for this setup.
Use this URL for the Submit button:
/lightning/action/quick/Order.Submit_new?context=RECORD_DETAIL&recordId={!CASESAFEID(Order.Id)}.
It retrieves the record ID, opens the 'Submit_new' action, and passes the record ID to it.
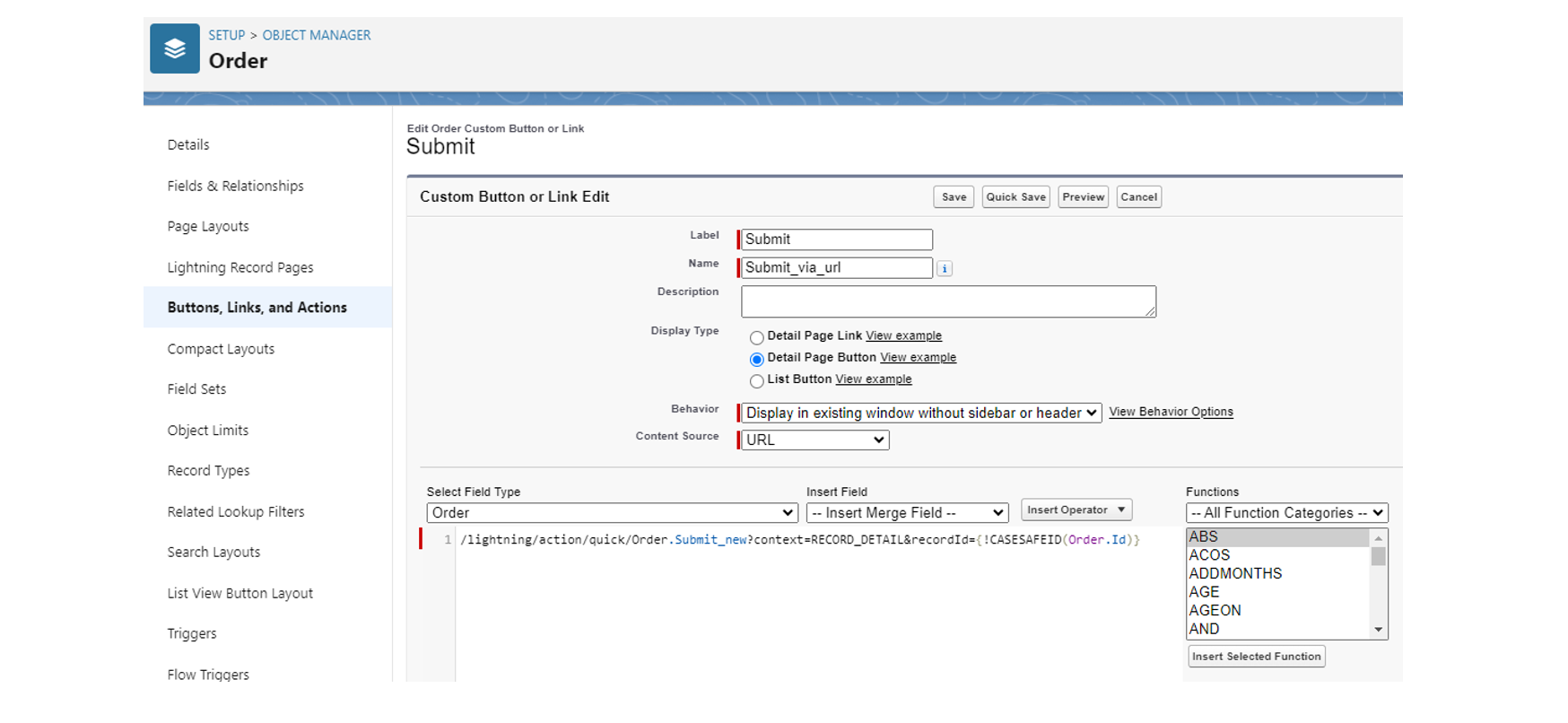
The Submit_new action simply runs the LWC component FirstBiometricService.
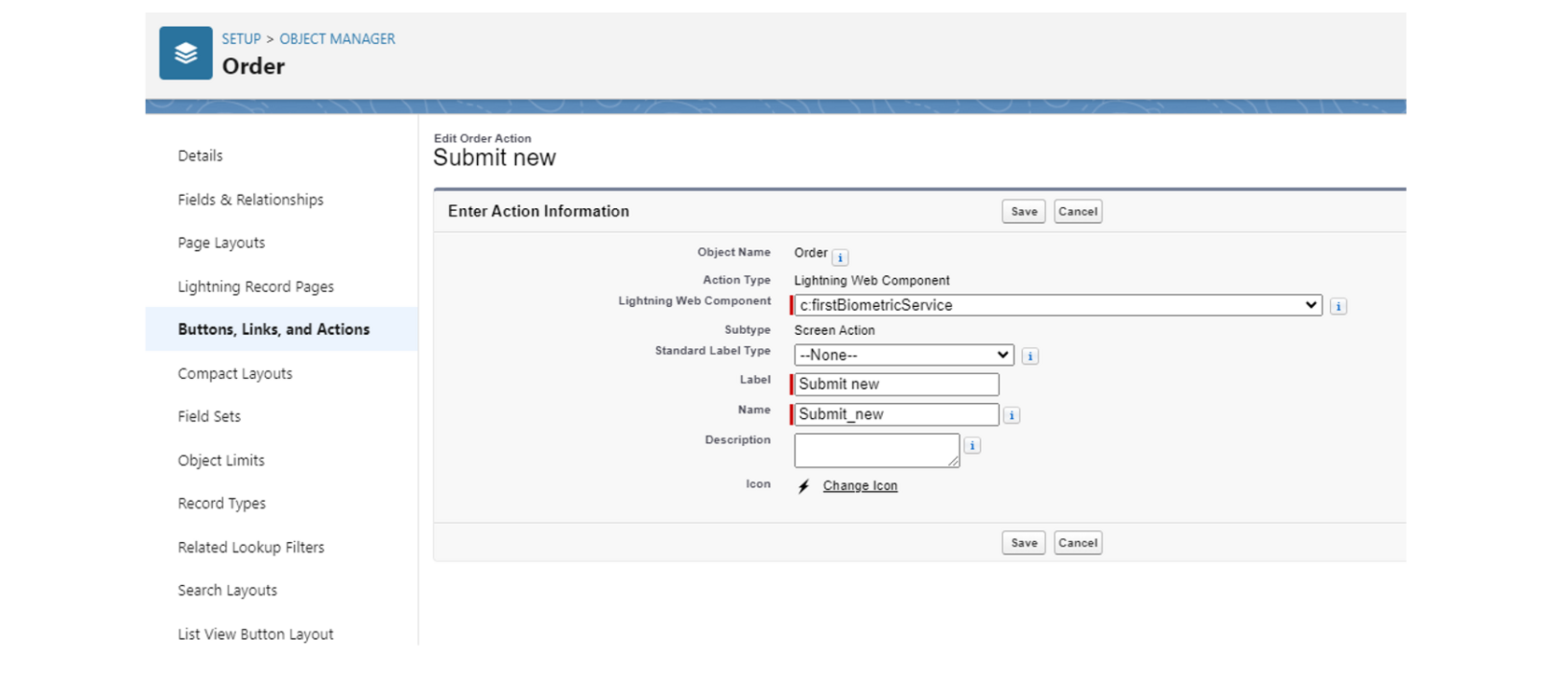
Next, just add Submit button to the Page Layout and Lightning Page with filter (just for mobile devices).
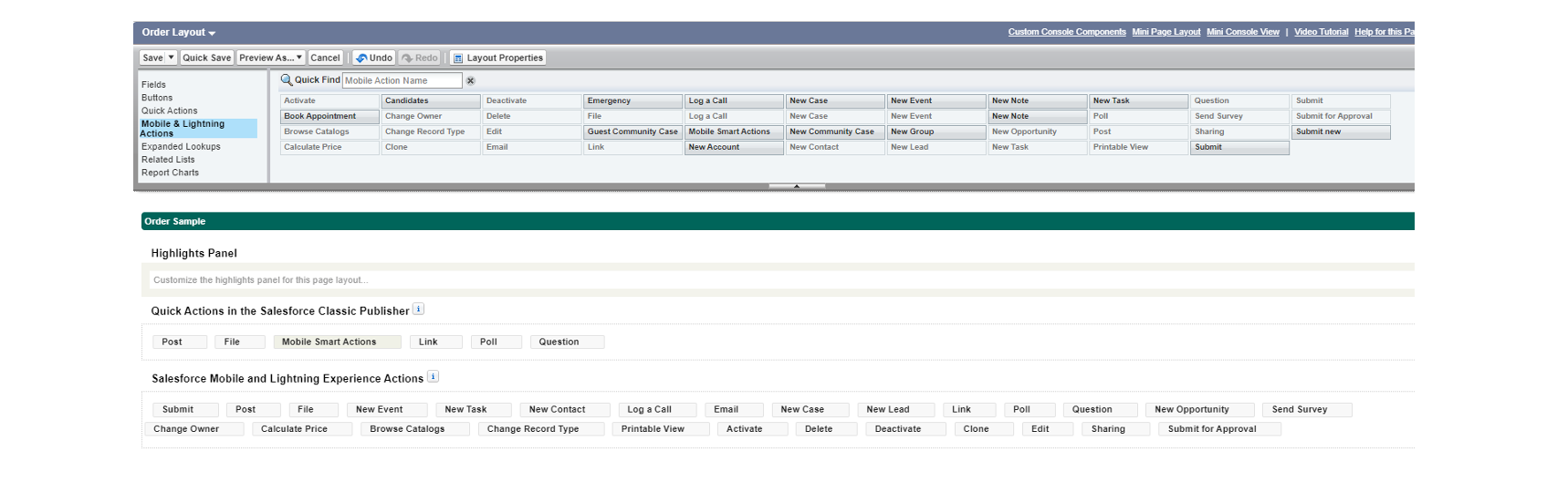
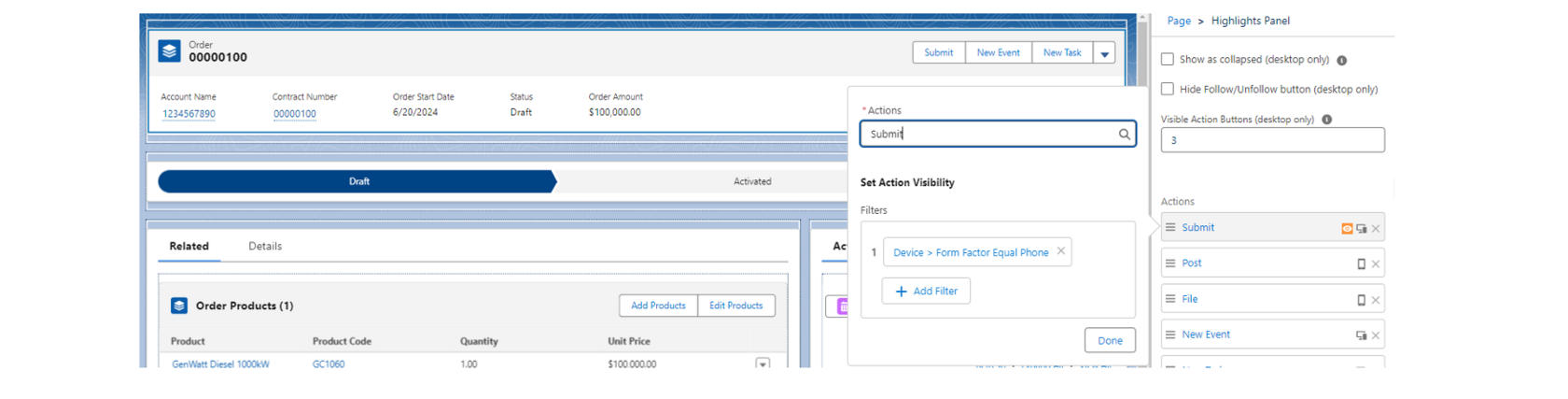
firstBiometricService.html
<template>
</template>
<template>
</template>

firstBiometricService.js
import {api, LightningElement, wire} from 'lwc';
import {getBiometricsService} from "lightning/mobileCapabilities";
import {updateRecord} from 'lightning/uiRecordApi';
import ID_FIELD from '@salesforce/schema/Order.Id';
import STATUS from '@salesforce/schema/Order.Status';
import {ShowToastEvent} from 'lightning/platformShowToastEvent';
export default class FirstBiometricService extends LightningElement {
biometricsService;
@api
recordId;
connectedCallback() {
this.biometricsService = getBiometricsService();
this.handleVerifyClick();
}
handleVerifyClick() {
if (this.biometricsService.isAvailable()) {
const options = {
permissionRequestBody: "Required to confirm device ownership.",
additionalSupportedPolicies: ['PIN_CODE']
};
this.biometricsService.checkUserIsDeviceOwner(options)
.then((result) => {
if (result === true) {
this.status = "✔ Current user is device owner."
const fields = {};
fields[ID_FIELD.fieldApiName] = this.recordId;
fields[STATUS.fieldApiName] = 'Activated';
const recordInput = { fields };
updateRecord(recordInput)
.then(() => {
this.dispatchEvent(
new ShowToastEvent({
title: 'Success',
message: 'Order submitted',
variant: 'success'
})
);
})
.catch(error => {
this.dispatchEvent(
new ShowToastEvent({
title: 'Error updating record',
message: this.recordId,
variant: 'error'
})
);
})
} else {
this.status = "𐄂 Current user is NOT device owner."
}
})
.catch((error) => {
this.status = 'Error code: ' + error.code + '\nError message: ' + error.message;
});
} else {
this.status = 'Problem initiating Biometrics service. Are you using a mobile device?';
}
}
}
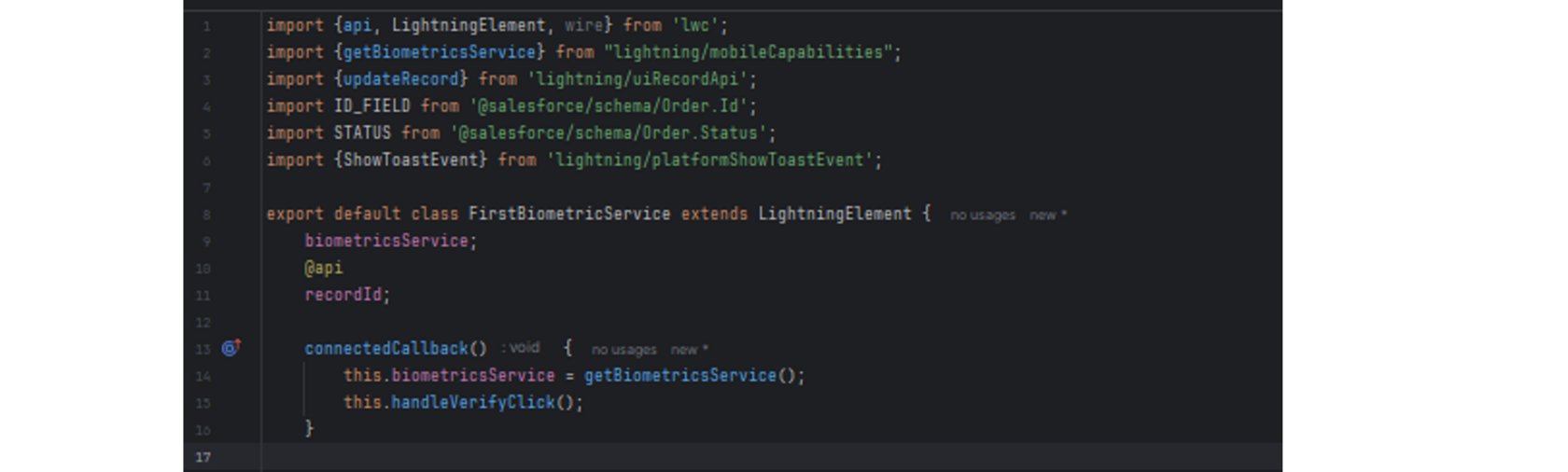
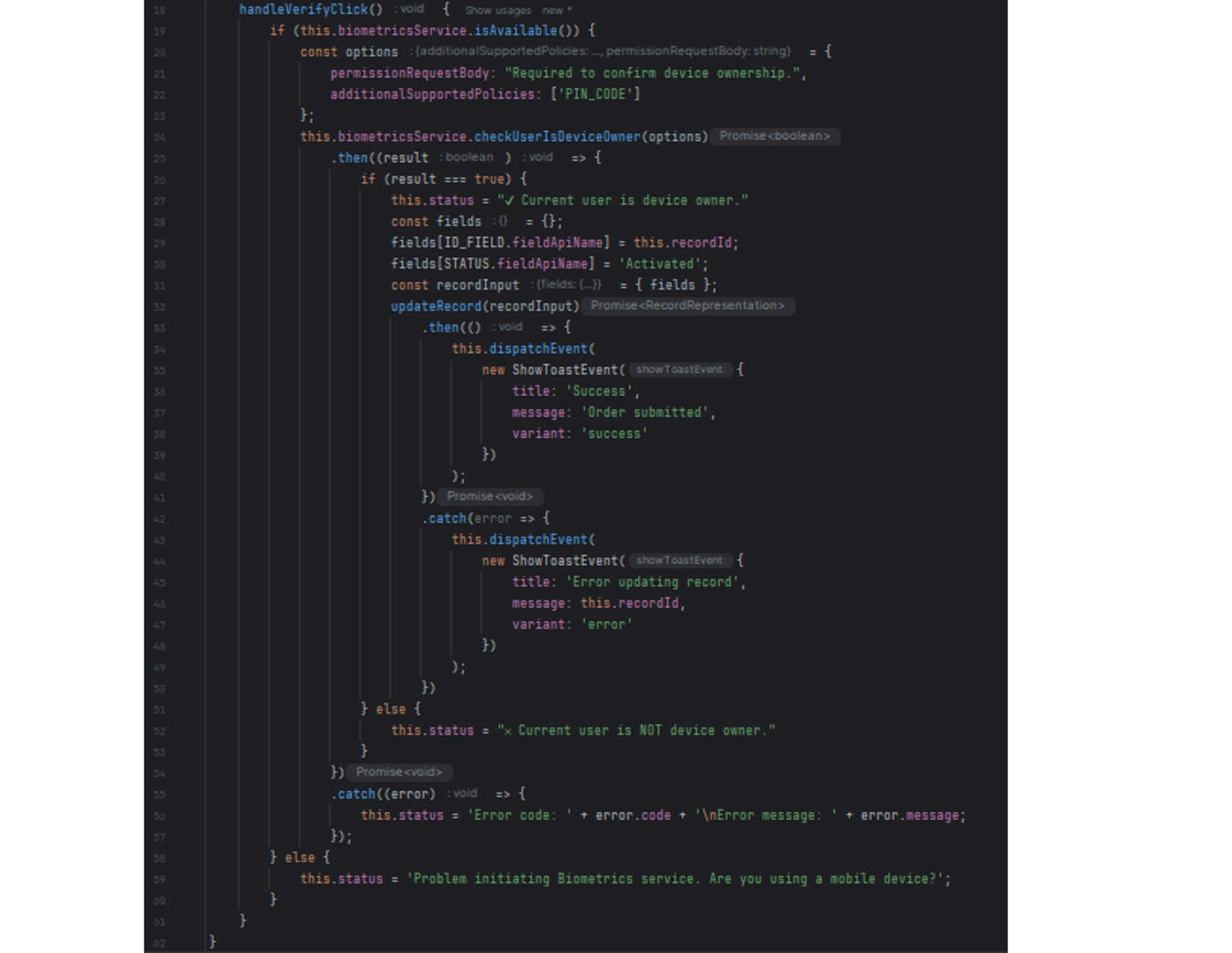
firstBiometricService.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>61.0</apiVersion>
<description>First Biometric Service</description>
<isExposed>true</isExposed>
<masterLabel>First Biometric Service</masterLabel>
<targets>
<target>lightning__AppPage</target>
<target>lightning__Tab</target>
<target>lightning__RecordPage</target>
<target>lightning__RecordAction</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordPage">
<supportedFormFactors>
<supportedFormFactor type="Small"/>
</supportedFormFactors>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
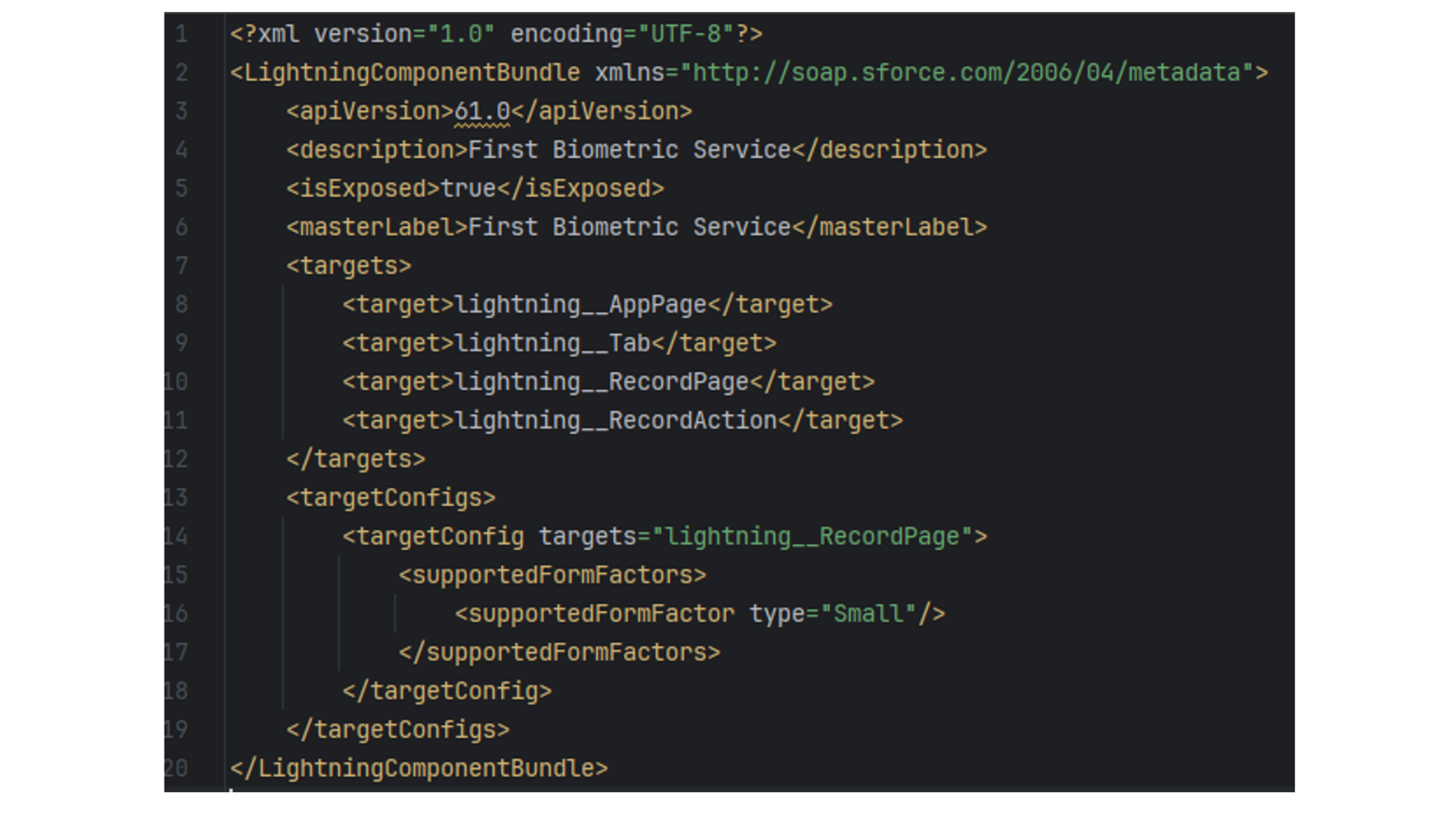
How It Works
Watch the demo:
video_usecase_example_sa_mobile_app.mp4
The Submit button's action is set to be visible only on mobile devices to prevent issues on desktop. Alternatively, you can handle this in the code by allowing record submission from desktop while running the BiometricsService on mobile.
Example 2:
Create an app page with an LWC displaying a table of secured records (e.g., Contacts) that only appears after passing the BiometricsService on mobile. This prevents unauthorized access if someone loses their device.
Watch the demo:
video_usecase_example_secured_table_in_mobile.mp4
However, the secured list can be viewed from a desktop without using the BiometricsService.
Watch the demo:
video_secure_table_in_desktop.mp4
Watch the demo:
video_usecase_example_sa_mobile_app.mp4
The Submit button's action is set to be visible only on mobile devices to prevent issues on desktop. Alternatively, you can handle this in the code by allowing record submission from desktop while running the BiometricsService on mobile.
Example 2:
Create an app page with an LWC displaying a table of secured records (e.g., Contacts) that only appears after passing the BiometricsService on mobile. This prevents unauthorized access if someone loses their device.
Watch the demo:
video_usecase_example_secured_table_in_mobile.mp4
However, the secured list can be viewed from a desktop without using the BiometricsService.
Watch the demo:
video_secure_table_in_desktop.mp4
Conclusion
Incorporating the Salesforce BiometricsService API into your applications represents a significant leap forward in enhancing security and user experience. By leveraging biometric authentication, you can safeguard sensitive data and streamline the login process, reducing reliance on traditional passwords. This API is seamlessly integrated with Salesforce Lightning Web Components, offering an efficient and localized biometric authentication solution.
Recommendations:
1) Adopt Best Practices: Ensure biometric features are used exclusively in mobile environments and always provide fallback authentication options.
2) Test Thoroughly: Validate your implementation across different devices and Salesforce environments to ensure compatibility and user experience.
3) Stay Updated: Keep abreast of updates and improvements to the BiometricsService API to leverage new features and maintain optimal security.
Utilizing the BiometricsService API not only strengthens your application's security but also enhances user convenience, paving the way for more secure and user-friendly digital experiences.
Recommendations:
1) Adopt Best Practices: Ensure biometric features are used exclusively in mobile environments and always provide fallback authentication options.
2) Test Thoroughly: Validate your implementation across different devices and Salesforce environments to ensure compatibility and user experience.
3) Stay Updated: Keep abreast of updates and improvements to the BiometricsService API to leverage new features and maintain optimal security.
Utilizing the BiometricsService API not only strengthens your application's security but also enhances user convenience, paving the way for more secure and user-friendly digital experiences.